Weather
The Weather system allows you to manage weather conditions effectively even when the environment is set to "Weather" in the Environment panel.
Summary
Importing the module
Yahaha provides a built-in module for managing the Weather System.
To import this module, use the following code:
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
Properties
Property | Type | Description |
---|---|---|
season | string | Sets the season for the scene (Spring, Summer, Autumn, Winter). |
weather | string | Sets the weather (Clear, Cloudy, Foggy, Overcast, Rain, Snow, Hail, Thunderstorm, Dust Storm). |
time | number | Sets the current time (0 to 24). |
cloudCover | number | Indicates cloudiness (0 to 1). |
precipitation | number | Sets the quantity of rain, snow, or hail (0 to 1). |
windStrength | number | Sets wind strength (0 to 1). |
fogIntensity | number | Controls fog density (0 to 1). |
fogColor | color | Sets the color of fog. |
sunriseTime | number | Sets sunrise time (0 to 24). |
sunsetTime | number | Sets sunset time (0 to 24). |
sunColor | color | Sets the color of sunlight. |
moonPhase | string | Sets the moon phase (NewMoon, WaxingCrescent, FirstQuarter, WaxingGibbous, FullMoon, WaningGibbous, ThirdQuarter, WaningCrescent). |
moonColor | color | Sets the color of moonlight. |
aurorasExist | boolean | Determines if auroras are present (only effective between sunset and sunrise). |
aurorasColor | color | Sets the color of auroras (only effective between sunset and sunrise). |
enableSound | boolean | Enables or disables weather-related sound effects. |
Methods
Sets all available settings in the Weather panel.
Changes the weather type to rain.
Changes the weather type to thunderstorms.
Changes the weather type to snow.
SetColorProperty(propertyName, propertyValue)
Sets the color property for fogColor, sunColor, moonColor, and aurorasColor. propertyName: string propertyValue: table, color value
SetStringProperty(propertyName, propertyValue)
Sets the string property for season, weather, and moonPhase. propertyName: string propertyValue: string
SetBoolProperty(propertyName, propertyValue)
Set the boolean property of aurorasExist and enableSound. propertyName: string propertyValue: boolean
SetNumberProperty(propertyName, propertyValue)
Set the number property of time, cloudCover, precipitation, windStrength, fogIntensity, sunriseTime, and sunsetTime.
- propertyName: string
- propertyValue: number
Properties
season
string. Sets the season for the scene between Spring, Summer, Autumn, and Winter.
weather
string. Sets the weather for the scene. Enter Clear, Cloudy, Foggy, Overcast, Rain, Snow, Hail, Thunderstorm, Dust Storm.
time
number. Sets the present time for the scene between 0 to 24.
cloudCover
number. Sets how cloudy it is. A higher value indicates more cloudy weather. Ranges from 0 to 1.
precipitation
number. Ranges from 0 to 1. Sets the quantity of rain, snow, and hail.
windStrength
number. Sets how strong the wind blows. A higher value indicates a stronger wind. Ranges from 0 to 1.
fogIntensity
number. Sets how dense the fog is. A higher value indicates more dense fog. Ranges from 0 to 1.
fogColor
Sets the color of fog.
sunriseTime
number. Sets the time at which the sun rises above the horizon. Ranges from 0 to 24.
sunsetTime
number. Sets the time at which the sun sets below the horizon. Ranges from 0 to 24.
sunColor
color. Sets the color of sunlight.
moonPhase
string. Sets the moon phrase between NewMoon, WaxingCrescent, FirstQuarter, WaxingGibbous, FullMoon, WaningGibbous, ThirdQuarter, and WaningCrescent.
moonColor
color. Sets the color of moonlight.
aurorasExist
boolean. Sets if auroras are present. Only works when the time is set between sunset and sunrise.
aurorasColor
color. Sets the color of auroras. Only works when the time is set between sunset and sunrise.
enableSound
Sets whether sound effects related to weather are active.
Methods
SetWeather
Sets all available settings in the Weather panel.
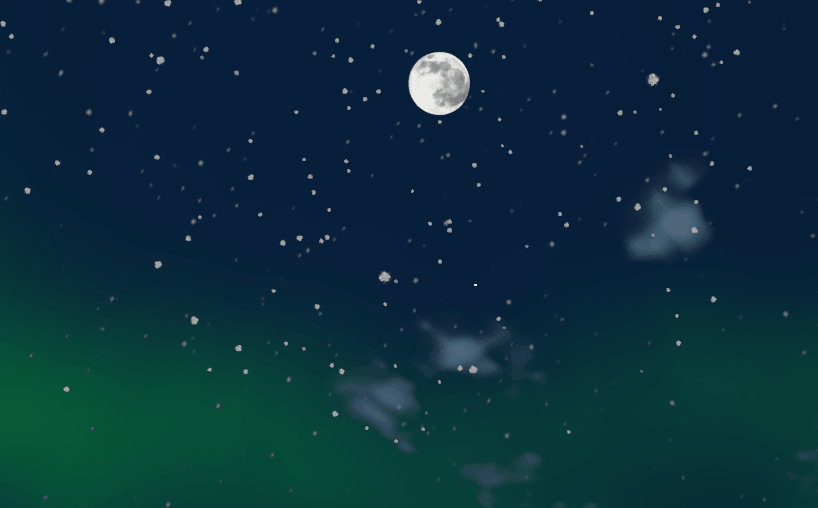
Code samples
The sample creates a dark midnight scene featuring flying snowflakes, neon green auroras, and a full moon.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
local weatherSetting =
{
season = "Winter",
weather = "Snow",
time = 23,
cloudCover = 0.3,
precipitation = 0.8,
windStrength = 0.3,
fogIntensity = 0,
fogColor = Color.New(1,1,1,1),
sunriseTime = 7.5,
sunsetTime = 17.5,
sunColor = Color.New(1,1,1,1),
moonPhase = "FullMoon",
moonColor = Color.New(1,1,1,1),
aurorasExist = true,
aurorasColor = Color.New(0,0.5,0,1)
}
weatherSystem.SetWeather(weatherSetting)
end)
ToRain
Changes the weather type to rain.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.ToRain()
end)
ToThunderstorm
Changes the weather type to thunderstorms.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.ToThunderstorm()
end)
ToSnow
Changes the weather type to snow.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.ToSnow()
end)
SetColorProperty(propertyName, propertyValue)
Sets the color property for fogColor, sunColor, moonColor, and aurorasColor.
- propertyName: string
- propertyValue: table, color value
Code samples
The sample demonstrates setting the sun color to solid green.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.SetColorProperty("sunColor", Color.New(0,1,0,1))
end)
SetStringProperty(propertyName, propertyValue)
Sets the string property for season, weather, and moonPhase.
- propertyName: string
- propertyValue: string
Code samples
The sample demonstrates setting the snowy weather.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.SetStringProperty("weather", "Snow")
end)
SetBoolProperty(propertyName, propertyValue)
Set the boolean property of aurorasExist and enableSound.
- propertyName: string
- propertyValue: boolean
To make auroras visible using aurorasExist
, make sure the time is set between sunset and sunrise.
Code samples
The code sample demonstrates adding auroras to the sky.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.SetBoolProperty("aurorasExist", true)
end)
SetNumberProperty(propertyName, propertyValue)
Set the number property of time, cloudCover, precipitation, windStrength, fogIntensity, sunriseTime, and sunsetTime.
- propertyName: string
- propertyValue: number
Code sample
This sample demonstrates how to set the scene's current time.
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
weatherSystem.SetNumberProperty("time", 24)
end)
StartUpdateTime(timeSpeed, targetTime)
Initiates the day-night cycle.
- timeSpeed: number. The default value is set to 1, which means that one second in the real world corresponds to one minute in the Weather System.
- targetTime: number. The default value is -1. When this value is set within the range of 0 to 24, the time updates will stop once it reaches the specified target time.
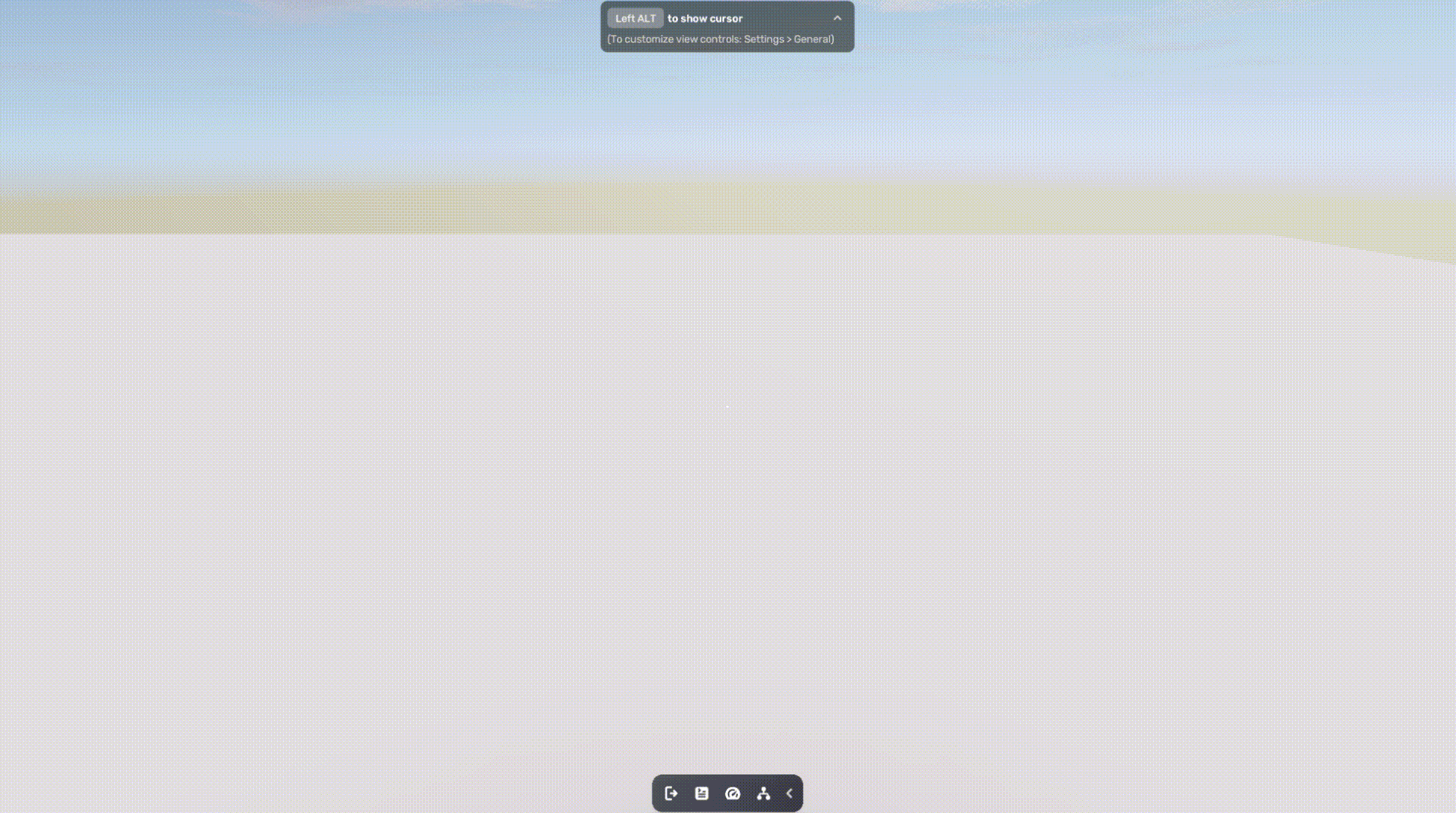
local weatherSystem = require("com.yahaha.sdk.graphics.WeatherUtils")
script.OnStart(function ()
-- Update time, one second in the real world equals 10 minutes in the game
weatherSystem.StartUpdateTime(10)
end)
StopUpdateTime()
Stops the day-night cycle.