Sky
The Sky system allows you to control various parameters of the sky and sunlight when the environment is set to "Sky" in the Environment panel.
Summary
Importing the module
Yahaha provides a built-in module for managing the Sky System.
To import this module, use the following code:
local skySystem = require("com.yahaha.sdk.graphics.SkyUtils")
Properties
Property | Type | Description |
---|---|---|
SunActive | boolean | Controls whether sunlight is active. |
SunBrightness | number | Controls sunlight brightness (0 to 10). |
SunColor | color | Controls the color of sunlight. |
SunRotation | Vector3 | Controls the rotation of the sun. |
SunShadowEnabled | boolean | Controls whether sunlight shadows are enabled. |
SunShadowIntensity | number | Controls shadow darkness (0 to 1). |
SkyRotation | number | Controls skybox rotation around its y-axis (0-360). |
SkyColor | color | Sets the color of the skybox. |
SkyExposure | number | Sets skybox visibility (0 to 1). |
SkyBrightness | number | Sets ambient light brightness (0 to 1). |
Properties
SunActive
boolean. Controls whether the sunlight is active. When set to true, the sunlight is visible; when set to false, the sunlight is disabled.
Code samples
The sample demonstrates how to turn off sunlight.
local skySystem = require("com.yahaha.sdk.graphics.SkyUtils")
script.OnStart(function ()
-- Turn off sunlight
skySystem.SunActive = false
end)
SunBrightness
number. Controls how bright the sunlight is. Higher values result in brighter sunlight. Ranges from 0 to 10 and defaults to 0.3.
Code samples The sample demonstrates how to control sunlight brightness.
local skySystem = require("com.yahaha.sdk.graphics.SkyUtils")
script.OnStart(function ()
-- Set sun brightness to zero.
skySystem.SunBrightness= 0
end)
SunColor
color. Controls the color of sunlight.
Code samples
The sample demonstrates how to make the sunlight color change gradually.
local skySystem = require("com.yahaha.sdk.graphics.SkyUtils")
script.OnUpdate(function ()
skySystem.SunColor = skySystem.SunColor + Color.New(0.002,0,0,0)
end)
SunRotation
Vector3. Controls the rotation of the sun.
Code samples The sample demonstrates a specified rotation of the sun.
local skySystem = require("com.yahaha.sdk.graphics.SkyUtils")
script.OnStart(function ()
-- Set the rotation of the sun.
skySystem.SunRotation = Vector3.New(0, 90, 0)
end)
SunShadowEnabled
boolean. Controls whether sunlight shadows are enabled. When set to true, shadows cast by sunlight are visible; when set to false, shadows are disabled.
SunShadowIntensity
number. Controls how dark sunlight shadows are. Higher values result in darker, more pronounced shadows. Ranges from 0 to 1.
SkyRotation
number. Controls the rotation of the skybox around its y-axis. Ranges from 0 to 360.
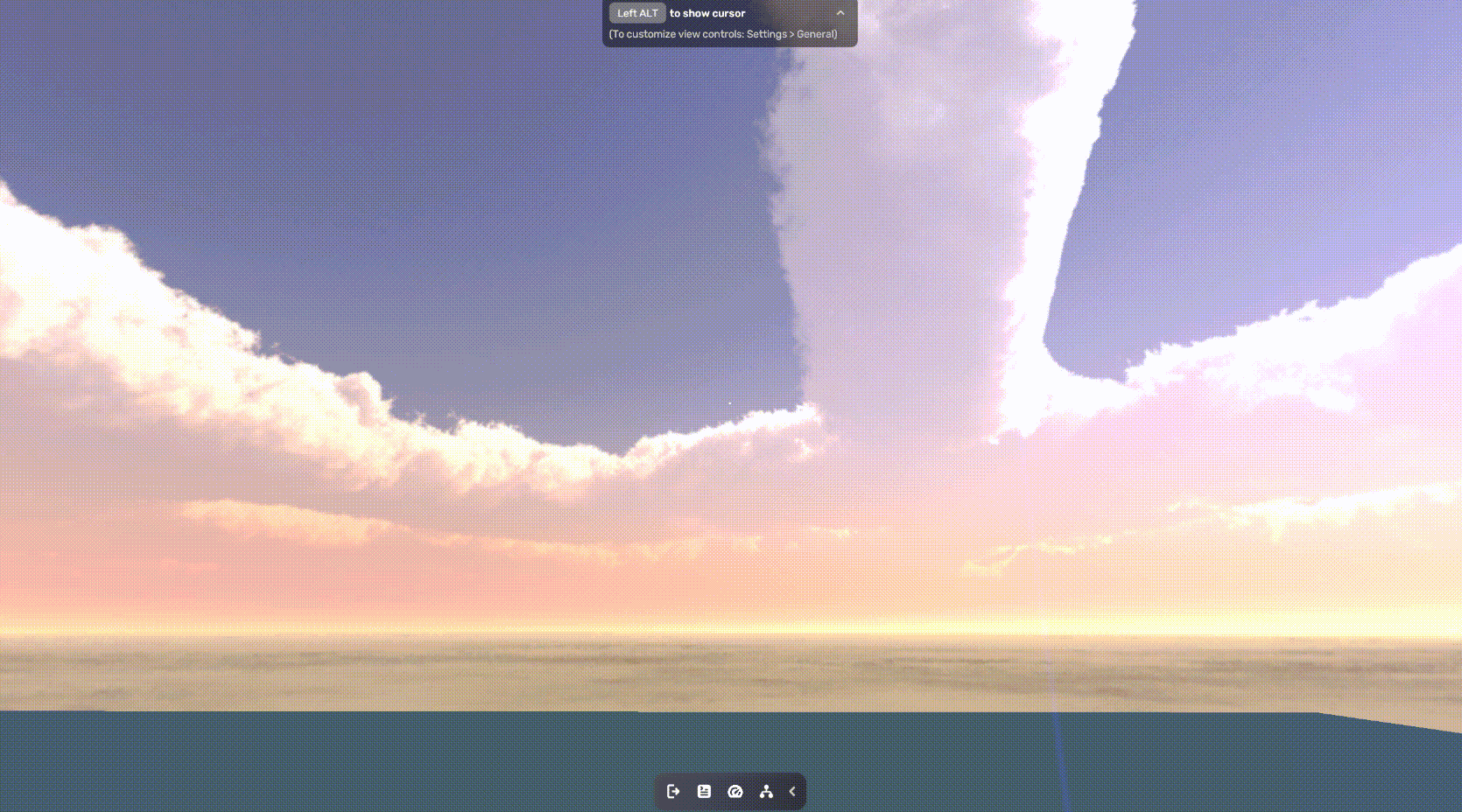
Code samples
The sample demonstrates how to rotate the skybox.
local skySystem = require("com.yahaha.sdk.graphics.SkyUtils")
script.OnUpdate(function ()
-- The skybox rotates around its y-axis one degree every frame
skySystem.SkyRotation = skySystem.SkyRotation + 1
end)
SkyColor
color. Sets the color of the skybox.
SkyExposure
number. Sets how much of the skybox is visible with a range from 0 (completely dark) to 1 (maximum brightness). Defaults to 0.13.
SkyBrightness
number. Sets how bright the ambient light is with a range from 0 (completely dark) to 1 (maximum brightness). Defaults to 0.13.