PostProcess
The PostProcess system allows for adding and editing post-processing effects through the Environment settings. This helps dramatically alter the appearance and mood of a scene without requiring additional geometry to be rendered.
Summary
Importing the module
Yahaha provides a built-in module for managing the PostProcess System.
To import this module, use the following code:
local PostProcessSystem = require("com.yahaha.sdk.graphics.PostProcessUtils")
Properties
Bloom
Property | Type | Description |
---|---|---|
Active | boolean | Enables or disables the bloom effect. |
Threshold | number | Brightness level for bloom activation (0 to 5). |
Intensity | number | Strength of the bloom effect (0 to 15). |
Color | color | Specifies the hue of the bloom effect. |
White Balance
Property | Type | Description |
---|---|---|
Active | boolean | Enables or disables white balance adjustments. |
Temperature | number | Adjusts color temperature (-1 to 1). |
Tint | color | Modifies scene tint (-1 to 1). |
Color Adjustments
Property | Type | Description |
---|---|---|
Active | boolean | Enables or disables color adjustments. |
PostExposure | number | Controls exposure level (0 to 5). |
Contrast | number | Adjusts image contrast (-1 to 1). |
ColorFilter | color | Applies a color filter across the scene. |
HueShift | number | Alters color hues (-180 to 180 degrees). |
Saturation | number | Modifies color saturation (-100 to 100). |
Vignette
Property | Type | Description |
---|---|---|
Active | boolean | Enables or disables the vignette effect. |
Intensity | number | Strength of the vignette effect (0 to 1). |
Color | color | Specifies the vignette's color. |
Smoothness | number | Adjusts transition smoothness (0 to 1). |
LiftGammaGain
Property | Type | Description |
---|---|---|
Active | boolean | Enables or disables LiftGammaGain adjustments. |
Lift | vector4 | Adjusts shadow colors. |
Gamma | vector4 | Modifies midtone colors. |
Gain | vector4 | Controls highlight colors. |
Fog
Property | Type | Description |
---|---|---|
Active | boolean | Enables or disables fog. |
Color | color | Specifies fog hue. |
FadeHeight | number | Height at which fog starts fading (-100 to 500). |
StartDistance | number | Distance from camera where fog begins appearing (0 to 500). |
Density | number | Thickness of fog (0 to 10). |
FadeSpeed | number | Speed at which fog fades out (0.01 to 0.5). |
Methods
SetPostProcessValue(postProcessName, propertyName, propertyValue)
Sets values for post-processing effects.
- postProcessName: string
- propertyName: string
- propertyValue: number/color/boolean/vector4
Post-process effects and their properties
Bloom
The bloom effect simulates the camera viewing a very bright light. It extends fringes from the border of bright areas to make them glow.
Properties
Active
boolean. Sets the bloom effect is active.
Threshold
number. Sets the brightness level at which the bloom effect begins to take effect. Ranges from 0 to 5.
Intensity
number. Controls the strength of the bloom effect. Ranges from 0 to 15, where lower values produce a subtle glow and higher values create a more pronounced and dramatic blooming effect.
Color
color. Specifies the color of the bloom effect, i.e., the glow's hue.
White Balance
White balance allows for adjustments to the color temperature and tint to achieve a more accurate color representation in the scene.
Properties
Active
boolean. Indicates whether the white balance adjustment is enabled (true) or disabled (false).
Temperature
number. Adjusts the color temperature of the scene, ranging from -1 to 1. Negative values shift the color towards cooler (bluer) tones, while positive values shift it towards warmer (redder) tones.
Tint
color. Modifies the tint of the scene, ranging from -1 to 1. Negative values introduce green hues, while positive values introduce magenta hues.
ColorAdjustments
ColorAdjustment modifies various color properties, enhancing the overall visual quality of the scene.
Properties
Active
boolean. Indicates whether color adjustments are enabled (true) or disabled (false).
PostExposure
number. Controls the exposure level applied to the scene after rendering, with a range from 0 to 5. Higher values increase brightness, making the image appear more illuminated, while lower values reduce brightness.
Contrast
number. Adjusts the contrast of the image, ranging from -1 to 1. Negative values decrease contrast, resulting in a flatter image, while positive values increase contrast, enhancing the distinction between light and dark areas.
ColorFilter
color. Applies a color filter to the entire scene. This property allows users to tint the image with a specific color, affecting the overall mood and atmosphere.
HueShift
number. Alters the hue of colors in the scene, with a range from -180 to 180 degrees. Negative values shift colors towards cooler tones, while positive values shift them towards warmer tones, enabling creative color manipulation.
Saturation
number. Modifies the saturation level of colors in the scene, ranging from -100 to 100. Negative values desaturate colors (making them more gray), while positive values enhance saturation (making colors more vivid).
Vignette
Vignette darkens the corners and edges of the image, drawing attention to the center of the frame.
Properties
Active
boolean. Indicates whether the vignette effect is enabled (true) or disabled (false).
Intensity
number. Controls the strength of the vignette effect, ranging from 0 (no vignette) to 1 (maximum darkening effect at the edges.)
Color
color. Specifies the color of the vignette effect.
Smoothness
number. Adjusts the transition between the vignette and the central area of the image, with a range from 0 to 1. Lower values create a sharper transition, while higher values produce a smoother, more gradual blend.
LiftGammaGain
LiftGammaGain allows for precise control over the tonal range of the image, affecting shadows, midtones, and highlights.
Properties
Active
boolean. Indicates whether the LiftGammaGain adjustments are enabled (true) or disabled (false).
Lift
vector4. Adjusts the lift (shadows) of the image. The xyz components range from 0 to 1, controlling the intensity of the shadow colors, while the w component ranges from -1 to 1, allowing for additional adjustment of shadow brightness. Lower values darken shadows, while higher values lighten them.
Gamma
vector4. Modifies the gamma (midtones) of the image. Similar to Lift, the xyz components range from 0 to 1, affecting midtone colors, and the w component ranges from -1 to 1 for further brightness adjustments. This property allows for fine-tuning of midtone brightness without significantly altering highlights or shadows.
Gain
vector4. Controls the gain (highlights) of the image. The xyz components range from 0 to 1, influencing highlight colors, while the w component ranges from -1 to 1 for additional highlight brightness adjustments. Increasing gain brightens highlights, while decreasing it darkens them.
Fog
Fog helps add depth and atmosphere to the scene.
Properties
Active
boolean. Indicates whether the fog effect is enabled (true) or disabled (false).
Color
color. Specifies the hue of the fog.
FadeHeight
number. Determines the height at which the fog begins to fade out, ranging from -100 to 500. A lower value means fog will start fading closer to the ground, while a higher value allows for fog to persist at greater heights.
StartDistance
number. Sets the distance from the camera at which the fog begins to appear, with a range from 0 to 500. This property helps control how quickly fog envelops the scene as it recedes into the distance.
Density
number. Controls the thickness of the fog, ranging from 0 to 10. Higher values result in denser fog, reducing visibility and creating a more pronounced atmospheric effect.
FadeSpeed
Number. Adjusts how quickly the fog fades out with distance, with a range from 0.01 to 0.5. Lower values create a slower fade, allowing for more gradual transitions, while higher values produce a quicker fade.
Methods
SetPostProcessValue(postProcessName, propertyName, propertyValue)
Sets values of post-processing effects.
Parameters:
- postProcessName: string
- propertyName: string
- propertyValue: number/color/boolean/vector4
Returns: void
Code samples
The sample demonstrates how to set the bloom effect.
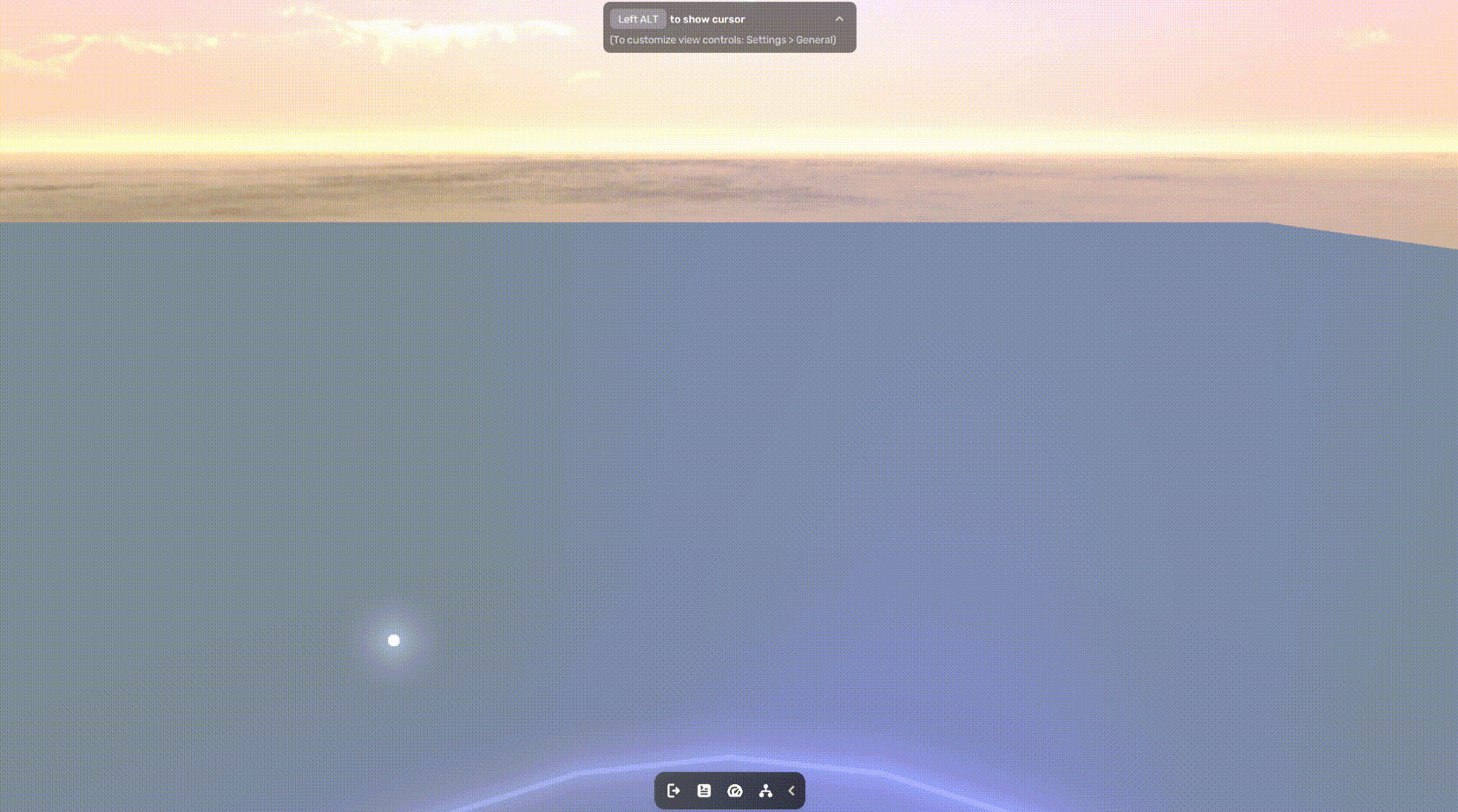
local PostProcessSystem = require("com.yahaha.sdk.graphics.PostProcessUtils")
script.OnStart(function ()
PostProcessSystem.SetPostProcessValue("Bloom", "Active", true)
PostProcessSystem.SetPostProcessValue("Bloom", "Intensity", 1)
PostProcessSystem.SetPostProcessValue("Bloom", "Color", Color.New(1,0,0,1))
end)
The following sample changes the LiftGammaGain post-processing effect in around 1 second.
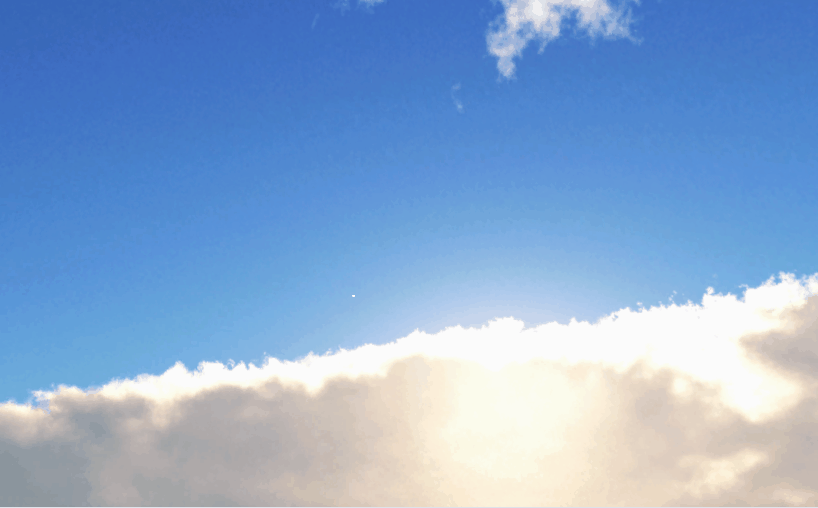
local PostProcessSystem = require("com.yahaha.sdk.graphics.PostProcessUtils")
local waitTime = 0
script.OnUpdate(function ()
waitTime = waitTime + 1
if waitTime < 200 then
return
end
PostProcessSystem.SetPostProcessValue("LiftGammaGain", "Active", true)
PostProcessSystem.SetPostProcessValue("LiftGammaGain", "Lift", Vector4.New(1,0,1,-0.5))
waitTime = 0
end)